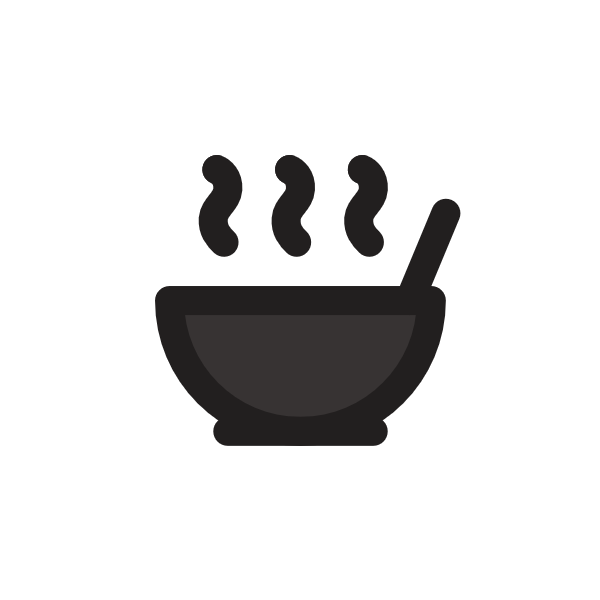
Cocojunk
🚀 Dive deep with CocoJunk – your destination for detailed, well-researched articles across science, technology, culture, and more. Explore knowledge that matters, explained in plain English.
Template metaprogramming
Read the original article here.
The Forbidden Code: Unleashing Compile-Time Power with Template Metaprogramming
Welcome, fellow code explorers, to the realm of forbidden techniques! Today, we delve into Template Metaprogramming (TMP), a powerful, often mind-bending practice that pushes the boundaries of what your compiler can do before your program even runs. Forget runtime tricks; we're talking about programming the compiler itself. This isn't your average classroom material, and for good reason – it can be complex, counter-intuitive, and sometimes feels more like solving logic puzzles than writing traditional code. But the rewards? Incredible compile-time performance, unparalleled code generation, and a deeper understanding of the compilation process.
What is Template Metaprogramming?
At its core, template metaprogramming (TMP) is a technique where you use the template system of a language to perform computations and generate code during the compilation phase, rather than at runtime.
Metaprogramming: Writing programs that manipulate other programs (or themselves) as their data. In the context of TMP, you write code (using templates) that the compiler executes to generate more code (or data structures, or constants) which then gets compiled along with the rest of your project.
Think of it this way: instead of your code calculating something when the user runs it, your code calculates it while the compiler is building the executable. The output of these compile-time computations, driven by templates, can be:
- Compile-time constants
- Generated data structures
- Entire functions or classes
The original intent of templates in languages like C++ was primarily for generic programming – writing functions or classes that work with various data types without repeating code (like std::vector
or std::sort
). However, sharp minds discovered that the rules governing how templates are defined, instantiated, and specialized created an unexpected side effect: a system capable of performing computations. This capability, unearthed somewhat by accident, became known as Template Metaprogramming.
While C++ is the most prominent language where TMP is discussed and heavily used (especially historically), similar compile-time code generation or manipulation facilities exist in other languages, sometimes through different mechanisms like powerful macro systems (think Lisp or Rust) or type-level programming. But C++ templates offer a unique, often intricate, form of compile-time execution.
The Engine Room: Components of Template Metaprogramming
Template metaprogramming isn't magic (though it can feel like it). It relies on the compiler's process of handling templates. To engage in TMP, you need two fundamental steps:
- Template Definition: You define a template (a blueprint) that describes the generic form of the code or calculation you want to perform at compile time. This definition might include template parameters (values or types the template operates on).
- Template Instantiation: You use or refer to the template with specific arguments (values or types). When the compiler encounters this usage, it instantiates the template, essentially executing the compile-time logic defined in the template using the provided arguments. This process generates the specific code or result needed.
The compiler then integrates the output of this instantiation into the final program before proceeding to the standard compilation steps.
Turing Completeness: Compiling Computations
One of the most fascinating aspects of template metaprogramming is its computational power.
Turing Completeness: A system is Turing-complete if it can simulate any single-taped Turing machine. In practical programming terms, a Turing-complete system can perform any computation that a standard computer program can, given enough time and memory.
Yes, you read that right. The C++ template system, originally designed for generic types, turned out to be a Turing-complete programming language in itself, running inside the compiler. This means, theoretically, any algorithm you can write in C++, Java, Python, etc., you could also express and compute using only C++ templates at compile time (though the syntax and methodology would be wildly different and often impractical for complex tasks).
TMP vs. Macros: A Fundamental Difference
It's crucial to distinguish template metaprogramming from another common compile-time technique: macros (especially the C preprocessor macros).
Macros: Textual or syntactic substitution tools that operate on source code before the main compilation phase.
- C/C++ Preprocessor Macros (
#define
): These are primarily textual substitution tools. They operate at a very low level, replacing text in your source code before the compiler even sees it properly parsed. They are independent of the language's syntax and type system, which makes them powerful but also dangerous (leading to unexpected side effects, difficult debugging, and lack of type safety). - Template Metaprogramming: Operates within the compiler's understanding of the language's syntax and type system. It's not just text replacement; it's a form of computation and code generation guided by the language's template rules. This awareness of types and structure makes TMP significantly more robust and type-safe than C preprocessor macros.
Think of it this way: macros are like a find-and-replace tool in a text editor operating on raw source code. TMP is like a specialized scripting language built into the compiler that understands your code's structure and types.
Functional Programming Paradigm at Compile Time
Interestingly, template metaprogramming, particularly in its older forms before modern C++ additions, often feels like functional programming.
Functional Programming: A programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing state and mutable data.
In TMP, template parameters and template-generated values are essentially immutable once determined during instantiation. There are no "variables" you can assign to and then change their values within the compile-time computation. Flow control is often achieved through recursion and template specialization (which acts like conditional logic or base cases for recursion) rather than traditional loops or if
statements operating on mutable state.
Harnessing the Compiler: Practical Uses of Template Metaprogramming
While its syntax can be alien, TMP has significant practical applications that justify its complexity in certain scenarios.
1. Generic Programming
This is the original and arguably most common use of templates, which TMP builds upon. TMP allows you to write code that works seamlessly with different types or values specified at compile time, avoiding code duplication.
- Basic Example: A template function
max<T>(T a, T b)
works forint
,float
, or any typeT
for which comparison (>
) is defined. The compiler generates specific versions (max<int>
,max<float>
, etc.) as needed. - TMP Extension: Beyond just handling types, TMP allows you to perform computations based on those types or based on compile-time values passed as template arguments, generating code tailored to specific needs. This moves beyond simple generic functions to more complex structure or algorithm generation.
2. Automatic Compile-Time Optimization
This is where TMP really shines in performance-critical code. By performing computations or generating code at compile time, you move work from the program's execution phase to its build phase.
Example: Compile-Time Factorial Calculation
A classic example is calculating factorials. A standard runtime recursive function looks like this:
// Traditional C++ (Runtime Calculation)
int factorial_runtime(int n) {
if (n == 0) {
return 1;
} else {
return n * factorial_runtime(n - 1);
}
}
// Usage:
int val1 = factorial_runtime(5); // Calculation happens when the program runs
int val2 = factorial_runtime(0); // Calculation happens when the program runs
With template metaprogramming, you can perform this calculation during compilation:
// C++ Template Metaprogramming (Compile-Time Calculation)
template<int N>
struct Factorial {
// Recursive step: inherits from Factorial<N-1> and calculates N!
static const int value = N * Factorial<N - 1>::value;
};
template<>
struct Factorial<0> {
// Base case for recursion: specialization for N=0
static const int value = 1;
};
// Usage:
// The compiler calculates Factorial<5>::value and Factorial<0>::value
// DURING compilation and substitutes the results (120 and 1) directly.
int val1 = Factorial<5>::value;
int val2 = Factorial<0>::value;
// This is equivalent to:
// int val1 = 120;
// int val2 = 1;
In this TMP example:
- The
template<int N> struct Factorial
defines the recursive step. Thevalue
is defined in terms ofFactorial<N-1>::value
. - The
template<> struct Factorial<0>
is a template specialization providing the base case, stopping the recursion. - When the compiler sees
Factorial<5>::value
, it needs to know what that is. It instantiatesFactorial<5>
. To getFactorial<5>::value
, it needsFactorial<4>::value
, which requiresFactorial<3>::value
, and so on, until it hits the specializationFactorial<0>
. It evaluatesFactorial<0>::value
(which is 1), thenFactorial<1>::value
(1 * 1), thenFactorial<2>::value
(2 * 1), ..., up toFactorial<5>::value
(5 * 24). - The final result (120) is computed by the compiler and placed directly into the compiled code as a constant, rather than calculating it every time the program runs.
Important Constraint: For this to work, the input to the template computation (N
in Factorial<N>
) must be known at compile time. This means N
must be a constant expression or a literal. You cannot use a variable whose value is only determined at runtime.
Modern C++ Alternatives (constexpr
, consteval
)
Since C++11, language features like constexpr
and later consteval
have been introduced, allowing certain functions to be evaluated at compile time if their inputs are constant expressions. This often provides a more readable syntax for compile-time value computation than the complex template-based methods for simple cases like factorial.
// Modern C++ (Compile-Time Calculation with constexpr/consteval)
constexpr int factorial_constexpr(int n) {
if (n == 0) {
return 1;
} else {
return n * factorial_constexpr(n - 1);
}
}
// Usage:
// If 5 and 0 are constant expressions, the compiler *can* evaluate
// factorial_constexpr(5) and factorial_constexpr(0) at compile time.
int val1 = factorial_constexpr(5);
int val2 = factorial_constexpr(0);
However, constexpr
/consteval
primarily handle value computation. Template metaprogramming retains its unique power for generating types, structures, and code based on compile-time inputs.
Example: Compile-Time Loop Unrolling
Another performance trick is loop unrolling. If the number of iterations is known at compile time, you can replace a loop with repeated code, potentially improving performance by reducing loop overhead (checking the condition, incrementing the counter, jumping). TMP can generate this unrolled code.
Consider adding two vectors of fixed, compile-time known length N
:
// Traditional Vector Addition (Runtime Loop)
void add_vectors_runtime(float* result, const float* a, const float* b, int length) {
for (int i = 0; i < length; ++i) {
result[i] = a[i] + b[i];
}
}
Using TMP, you could define a vector class template where the size is a template parameter:
// Template-based Fixed-Size Vector (Compile-time size)
template<typename T, int N>
struct Vec {
T data[N];
// Define addition using template recursion for unrolling
template<int I>
struct Adder {
// Recursive step: add element I, then add element I-1
static void add(T* res, const T* v1, const T* v2) {
res[I] = v1[I] + v2[I];
Adder<I - 1>::add(res, v1, v2); // Recurse
}
};
// Base case for recursion
template<>
struct Adder<0> {
static void add(T* res, const T* v1, const T* v2) {
res[0] = v1[0] + v2[0]; // Add the first element
}
};
// Addition operator using the compile-time adder
Vec<T, N> operator+(const Vec<T, N>& other) const {
Vec<T, N> result;
// Start the compile-time recursive addition
Adder<N - 1>::add(result.data, this->data, other.data);
return result;
}
};
// Usage:
Vec<float, 4> v1 = {1.0f, 2.0f, 3.0f, 4.0f};
Vec<float, 4> v2 = {5.0f, 6.0f, 7.0f, 8.0f};
Vec<float, 4> v_sum = v1 + v2; // The addition code is unrolled at compile time!
When the compiler instantiates Vec<float, 4>::operator+
, it instantiates Vec<float, 4>::Adder<3>::add
. This recursively calls Adder<2>::add
, then Adder<1>::add
, then Adder<0>::add
. The compiler effectively generates code equivalent to:
// Compiler-generated unrolled code for Vec<float, 4>::operator+
Vec<float, 4> operator+(const Vec<float, 4>& other) const {
Vec<float, 4> result;
result.data[3] = this->data[3] + other.data[3];
result.data[2] = this->data[2] + other.data[2];
result.data[1] = this->data[1] + other.data[1];
result.data[0] = this->data[0] + other.data[0];
return result;
}
This eliminates the runtime loop overhead.
Caution: Code Bloat: While beneficial for small fixed sizes, this technique can lead to "code bloat." If you use Vec<float, 4>
, Vec<float, 5>
, and Vec<int, 4>
, the compiler generates separate, unrolled code for the addition operation for each unique template instantiation. For many different sizes or types, this can significantly increase the size of your compiled executable.
3. Static Polymorphism (Compile-Time Dispatch)
Polymorphism, the ability to treat objects of different classes in a uniform way (usually via a common base class), is typically a runtime feature achieved with virtual functions and virtual tables (v-tables). This involves a small overhead for the runtime lookup.
Dynamic Polymorphism: Polymorphic behavior (determining which method implementation to call) is resolved at runtime, typically using virtual functions and v-tables.
Static Polymorphism: Polymorphic behavior is resolved at compile time, avoiding the runtime overhead of v-tables. This is often achieved using template techniques.
Template metaprogramming allows you to mimic polymorphism, but with the method dispatch resolved entirely at compile time. A key technique for this is the Curiously Recurring Template Pattern (CRTP).
Curiously Recurring Template Pattern (CRTP): A class
Base
takes its derived classDerived
as a template parameter:template <class Derived> class Base { ... }
. The base class can then use facilities of the derived class via astatic_cast
to theDerived
type.
This pattern allows you to define common functionality in the base class template and have it operate on or call methods of the derived class, effectively injecting base class behavior into the derived class at compile time.
// Example: CRTP for Static Polymorphism
template <typename Derived>
class BaseRenderer {
public:
// Common rendering logic
void render() const {
// Static cast 'this' (BaseRenderer<Derived>*) to Derived*
// and call a method expected to be in Derived
static_cast<const Derived*>(this)->draw();
}
// Other common methods...
};
// Derived classes inherit from the base template, passing themselves
class Square : public BaseRenderer<Square> {
public:
// Derived class provides the specific implementation
void draw() const {
std::cout << "Drawing a Square" << std::endl;
}
};
class Circle : public BaseRenderer<Circle> {
public:
// Derived class provides the specific implementation
void draw() const {
std::cout << "Drawing a Circle" << std::endl;
}
};
// Usage:
Square s;
Circle c;
s.render(); // Calls BaseRenderer<Square>::render(), which calls Square::draw()
c.render(); // Calls BaseRenderer<Circle>::render(), which calls Circle::draw()
// No virtual functions needed, dispatch resolved at compile time.
// You cannot store Square and Circle pointers in a single BaseRenderer* array
// like you would with dynamic polymorphism. The "polymorphism" is in the pattern,
// not the ability to treat instances uniformly via a base pointer/reference.
In this example, the render()
method is defined once in BaseRenderer
. Because BaseRenderer
is templated on the Derived
type, the compiler generates a specific version of BaseRenderer
(and its methods like render()
) for each derived class (Square
and Circle
). Inside BaseRenderer<Square>::render()
, the static_cast<const Square*>(this)
is valid, and the call static_cast<const Square*>(this)->draw()
resolves directly to Square::draw()
at compile time. The same happens for BaseRenderer<Circle>::render()
, resolving to Circle::draw()
.
This avoids the overhead of dynamic dispatch but means you lose the ability to easily work with a collection of objects of different derived types through a common base pointer. Static polymorphism is useful when the type is known at compile time.
Related to CRTP is the "Barton–Nackman trick" or "restricted template expansion," where a base class template provides common implementation details that derived classes must use, often enforcing behavior or structure implicitly rather than through explicit contracts like interfaces.
4. Static Table Generation
Look-up tables are a common optimization technique to replace potentially slow computations with fast array indexing. TMP can be used to calculate the values for these tables at compile time, baking them directly into the executable as constants.
Lookup Table: An array or map used to replace runtime computation with a simple data lookup.
Here's an example generating a table of squares at compile time using TMP recursion and variadic templates (C++11/14 style):
// C++11/14 Style Static Table Generation (Squares)
template <int... N> // Variadic template parameters
struct Sequence {}; // Empty struct to help with recursion
// Recursive struct to build the sequence of squares
template <int N, int... Rest>
struct SquaresHelper : SquaresHelper<N - 1, N * N, Rest...> {}; // Add N*N and recurse
// Base case: Stop recursion when N reaches 0
template <int... Rest>
struct SquaresHelper<0, Rest...> {
// This specialization uses the accumulated sequence (Rest)
typedef Sequence<0 * 0, Rest...> type; // Add 0*0 and store the sequence
};
// Struct to hold the generated table
template <int... N>
struct StaticSquaresTable {
// Use the generated sequence to initialize the array
static const int values[sizeof...(N)] = {N...};
};
// Putting it together: Generate a table of squares up to 9
// SquaresHelper<9> -> SquaresHelper<8, 9*9> -> SquaresHelper<7, 8*8, 9*9> -> ...
// -> SquaresHelper<0, 1*1, ..., 9*9> -> Sequence<0*0, 1*1, ..., 9*9>
typedef SquaresHelper<9>::type SquaresSequence;
// Instantiate the table with the generated sequence
template struct StaticSquaresTable<SquaresSequence::N...>;
// Access the table:
// The compiler will generate an array like:
// const int StaticSquaresTable_values[] = {0, 1, 4, 9, 16, 25, 36, 49, 64, 81};
// (Assuming INDEX goes from 0 up to SIZE-1)
const int* squares_table = StaticSquaresTable<SquaresSequence::N...>::values;
// Example usage:
// int square_of_5 = squares_table[5]; // Access pre-calculated value (25)
This older approach is complex because it uses template recursion to build a sequence of numbers (the squares) that is then unpacked by a variadic template into an array initialization.
With C++17, constexpr
lambdas and if constexpr
(along with enhanced constexpr
capabilities) often make static table generation much simpler and more readable:
// C++17 Style Static Table Generation (Squares)
template<int Size>
struct StaticSquaresTableC17 {
static constexpr int values[Size] = [] { // Use a constexpr lambda for initialization
int table[Size];
for (int i = 0; i < Size; ++i) { // Use a standard loop (allowed in constexpr lambda)
table[i] = i * i;
}
return table; // Return the populated array
}(); // Immediately invoke the lambda
// C++20 allows constexpr for std::vector, offering even more flexibility
};
// Instantiate and access the table
const int* squares_table_c17 = StaticSquaresTableC17<10>::values;
// Example usage:
// int square_of_5 = squares_table_c17[5]; // Access pre-calculated value (25)
The C++17 approach is much closer to traditional C++ syntax while achieving the same compile-time computation and table generation. However, it still requires the size (Size
) to be a compile-time constant. The older, more complex template-recursive methods might still be seen in legacy code or for patterns not easily expressed with constexpr
functions alone.
5. Concepts (C++20): Guiding Template Instantiation
While older TMP could achieve powerful results, its error messages were notoriously difficult to decipher (often involving complex template instantiation traces). C++20 introduced Concepts as a way to explicitly specify requirements for template arguments, making template code more readable and enabling better error messages.
Concepts: Named sets of requirements (syntactic and semantic) for template parameters. Compilers use concepts during template matching to determine if a type is suitable for a template, improving type checking and error reporting.
Concepts aren't strictly a TMP technique in the sense of compile-time computation, but they are a crucial tool for compile-time template programming, allowing you to control and constrain the template instantiation process in a structured way. They influence which template specialization the compiler chooses based on type properties, which can be part of complex compile-time logic.
Here's the FizzBuzz example mentioned in the source, demonstrating how concepts can guide template selection based on compile-time divisibility checks:
// C++20 Concepts Example: FizzBuzz at Compile Time
#include <iostream>
// Define concepts for divisibility
template<int N>
concept DivisibleBy3 = (N % 3) == 0;
template<int N>
concept DivisibleBy5 = (N % 5) == 0;
// Template structure to handle FizzBuzz logic based on concepts
template<int N>
struct FizzBuzzPrinter {
// Default case: print the number
static void print() {
std::cout << N << std::endl;
}
};
// Specialization for numbers divisible by both 3 and 5
template<int N> requires DivisibleBy3<N> && DivisibleBy5<N>
struct FizzBuzzPrinter<N> {
static void print() {
std::cout << "FizzBuzz" << std::endl;
}
};
// Specialization for numbers divisible by 3 (but not 5, due to order/requires)
template<int N> requires DivisibleBy3<N>
struct FizzBuzzPrinter<N> {
static void print() {
std::cout << "Fizz" << std::endl;
}
};
// Specialization for numbers divisible by 5 (but not 3, due to order/requires)
template<int N> requires DivisibleBy5<N>
struct FizzBuzzPrinter<N> {
static void print() {
std::cout << "Buzz" << std::endl;
}
};
// Template recursion to print FizzBuzz for a range
template<int Current, int End>
struct FizzBuzzRange {
static void print() {
FizzBuzzPrinter<Current>::print(); // Print for the current number
// Recurse to the next number
if constexpr (Current < End) { // Use if constexpr for compile-time branching
FizzBuzzRange<Current + 1, End>::print();
}
}
};
// Usage: Print FizzBuzz from 1 to 15 at compile time
// FizzBuzzRange<1, 15>::print()
// This expands into calls like:
// FizzBuzzPrinter<1>::print();
// FizzBuzzPrinter<2>::print();
// FizzBuzzPrinter<3>::print(); // selects 'Fizz' specialization
// ...
// FizzBuzzPrinter<15>::print(); // selects 'FizzBuzz' specialization
// etc.
int main() {
FizzBuzzRange<1, 15>::print();
return 0;
}
In this example:
DivisibleBy3<N>
andDivisibleBy5<N>
are concepts that check a compile-time property (N % 3 == 0
orN % 5 == 0
).- The compiler uses these concepts in the
requires
clause of the template specializations forFizzBuzzPrinter
. When you requestFizzBuzzPrinter<15>::print()
, the compiler checks which specialization's requirements are met.requires DivisibleBy3<15> && DivisibleBy5<15>
is true, so it selects theFizzBuzz
specialization. ForFizzBuzzPrinter<9>::print()
, onlyrequires DivisibleBy3<9>
is true, selecting theFizz
specialization (theFizzBuzz
specialization is preferred when its requirements are met). FizzBuzzRange
uses template recursion andif constexpr
(another C++17/20 feature allowing compile-time conditional branching) to generate the sequence of calls toFizzBuzzPrinter
.
This demonstrates how concepts, combined with other modern C++ features, allow for more structured and expressive compile-time logic compared to older, less readable TMP idioms based purely on recursion and specialization order (which implicitly handled conditions like "if divisible by 3 AND 5" by having that specialization be more specific and thus preferred).
The Tradeoffs: Benefits and Drawbacks
Like any powerful technique, TMP comes with its own set of pros and cons.
Benefits:
- Performance: By shifting computation and code generation to compile time, you eliminate runtime overhead, potentially leading to significant performance gains in specific areas (like the unrolled loop example).
- Flexibility and Genericity: TMP allows for incredibly flexible and highly specialized code to be generated based on compile-time inputs, achieving a level of genericity beyond what's easily possible with runtime techniques.
- Code Minimization & Maintainability (in specific ways): Although TMP syntax can be complex, the definitions can be written once and then generate vast amounts of tailored code. This can reduce repetitive manual coding, making maintenance easier if you understand the TMP code itself.
- Enforcing Constraints at Compile Time: TMP can be used to perform checks or enforce constraints on types or values before the program even compiles. If a constraint is violated, you get a compile-time error rather than a runtime crash.
Drawbacks:
- Complexity and Readability (Historically): For a long time (pre-C++11/14/17/20), TMP syntax was famously arcane and difficult to read and write. It required thinking in terms of recursive type structures and template specializations as flow control. While modern C++ features like
constexpr
and concepts have improved the situation for certain tasks, complex TMP still requires a deep understanding of the template system. - Compile Time: Performing significant computations and code generation at compile time can drastically increase your program's compilation time. This can slow down development iteration cycles.
- Error Messages: Historically, TMP compile errors were legendary for their length and obscurity, showing deep dives into template instantiation layers. Concepts have helped, but complex TMP errors can still be challenging to debug.
- Code Bloat: As seen with the vector unrolling example, generating specialized code for many different template instantiations can significantly increase the size of the final executable.
- Difficulty Debugging: Since the code runs at compile time, traditional runtime debuggers are useless for stepping through TMP logic. Debugging often relies on carefully reading the code, using compiler-specific features, or sometimes deliberately causing compile errors to inspect template instantiation paths.
Conclusion: Mastering the Forbidden Arts
Template metaprogramming is a deep, powerful technique that transforms your compiler from a passive translator into an active participant in computation and code generation. It's not always necessary, and its complexity often means it should be reserved for specific problems where the benefits (like compile-time performance or static code generation) outweigh the costs in development time and code readability.
Understanding TMP is like learning a secret language within C++. It unlocks the ability to perform "forbidden" compile-time hacks that can give your code a significant edge in performance and flexibility, particularly in areas like generic libraries, high-performance computing, and embedded systems where every clock cycle and byte counts. While modern C++ offers more approachable ways to do some compile-time tasks, the core principles and older patterns of TMP remain fundamental to understanding advanced C++ and the power hidden within its template system. Dive deep, practice, and you'll gain a perspective on compilation and code generation they definitely won't teach you in a standard introductory course!